Web服务器
实验要求
将HTML文件(例如HelloWorld.html)放在服务器所在的目录中。运行服务器程序。确认运行服务器的主机的IP地址(例如128.238.251.26)。从另一个主机,打开浏览器并提供相应的URL。例如:
http://128.238.251.26:6789/HelloWorld.html
“HelloWorld.html”是您放在服务器目录中的文件。还要注意使用冒号后的端口号。您需要使用服务器代码中使用的端口号来替换此端口号。在上面的例子中,我们使用了端口号6789. 浏览器应该显示HelloWorld.html的内容。如果省略“:6789”,浏览器将使用默认端口80,只有当您的服务器正在端口80监听时,才会从服务器获取网页。
然后用客户端尝试获取服务器上不存在的文件。你应该会得到一个“404 Not Found”消息。
代码
1 | #import socket module |
UDP Ping程序
实验要求
在本实验中,您将学习使用Python进行UDP套接字编程的基础知识。您将学习如何使用UDP套接字发送和接收数据报,以及如何设置适当的套接字超时。在实验中,您将熟悉Ping应用程序及其在计算统计信息(如丢包率)中的作用。
您首先需要研究一个用Python编写的简单的ping服务器程序,并实现对应的客户端程序。这些程序提供的功能类似于现代操作系统中可用的标准ping程序功能。然而,我们的程序使用更简单的UDP协议,而不是标准互联网控制消息协议(ICMP)来进行通信。 ping协议允许客户端机器发送一个数据包到远程机器,并使远程机器将数据包返回到客户(称为回显)的操作。另外,ping协议允许主机计算它到其他机器的往返时间。
代码
1 | # UDPPingerServer.py |
1 | from socket import * |
邮件客户端
实验要求
创建一个向任何接收方发送电子邮件的简单邮件客户。你的客户将必须与邮件服务器(如谷歌的电子邮件服务器)创建一个TCP连接,使用SMTP协议与该邮件服务器进行交谈,经该邮件服务器向某接收方(如你的朋友)发送一个电子邮件报文,最后关闭与该邮件服务器的TCP连接。
本实验参考:https://blog.csdn.net/JavaMoo/article/details/54897033
代码
1 | from socket import * |
代理服务器
实验要求
在本实验中,您将了解Web代理服务器的工作原理及其基本功能之一 —— 缓存。
您的任务是开发一个能够缓存网页的小型Web代理服务器。这是一个很简单的代理服务器,它只能理解简单的GET请求,但能够处理各种对象 —— 不仅仅是HTML页面,还包括图片。
通常,当客户端发出一个请求时,请求将被直接发送到Web服务器。然后Web服务器处理该请求并将响应消息发送客户端。为了提高性能,我们在客户端和Web服务器之间建立一个代理服务器。现在,客户端发送的请求消息和Web服务器返回的响应消息都要经过代理服务器。换句话说,客户端通过代理服务器请求对象。代理服务器将客户端的请求转发到Web服务器。然后,Web服务器将生成响应消息并将其传递给代理服务器,代理服务器又将其发送给客户端。
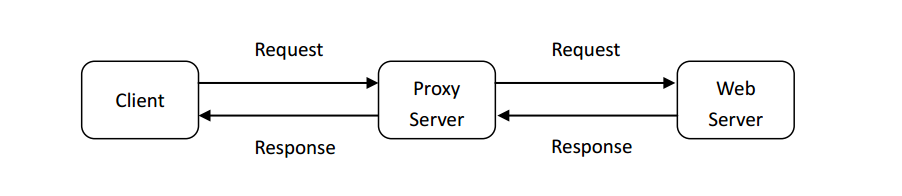
代码
1 | from socket import * |
ICMP Ping程序
实验要求
在这个作业中,您将更好地理解因特网控制报文协议(ICMP)。您会学习使用ICMP请求和响应消息实现Ping程序。
Ping是一个网络应用程序,用于测试某个主机在IP网络中是否可访问。它也用于测试计算机的网卡或测试网络延迟。它通过向目标主机发送ICMP“回显”包并监听ICMP“回显”应答来工作。“回显”有时称为"pong"。ping程序测量往返时间,记录数据包丢失,并输出接收到的回显包的统计摘要(往返时间的最小值、最大值和平均值,以及在某些版本中的平均值的标准差)。
您的任务是用python开发自己的Ping程序。您的程序将使用ICMP,但为了保持简单,将不完全遵循RFC 1739中的正式规范。请注意,您只需要编写程序的客户端,因为服务器端所需的功能几乎内置于所有操作系统中。
您的Ping程序能将ping请求发送到指定的主机,间隔大约一秒钟。每个消息包含一个带有时间戳的数据包。每个数据包发送完后,程序最多等待一秒,用于接收响应。如果一秒后服务器没有响应,那么客户端应假设ping数据包或pong数据包在网络中丢失(或者服务器已关闭)。
代码
1 | import socket |
本着互联网开源的性质,欢迎分享这篇文章,以帮助到更多的人,谢谢!